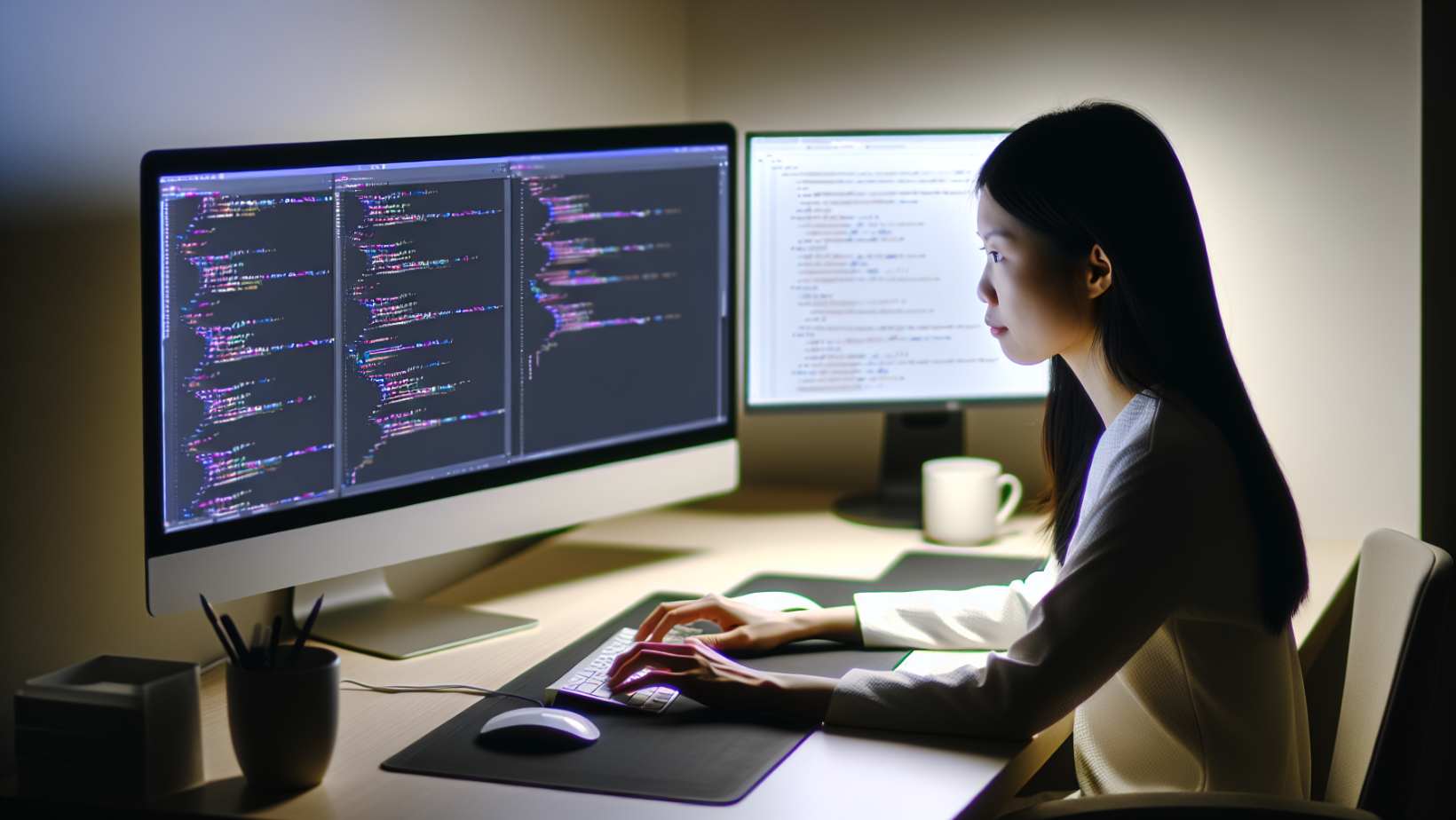
Are you curious about the wizardry that enables websites to offer interactive features or react to your every click? Look no further – it’s JavaScript in action! “What is JavaScript? A Beginner’s Guide to the Basics of JS” is crafted to help you grasp the foundations of this vital programming language, from simple actions to complex applications.
We’ll walk you through what JavaScript is, its functionality, and its pivotal role in modern web development, giving you the building blocks to start your own script-writing adventure.
Decoding JavaScript: The Language of the Web
Have you ever wondered what powers the interactive elements on your favorite websites? The animations that make your browsing experience dynamic and engaging? That’s the magic of JavaScript, a scripting language that brings web pages to life.
Introduced in 1995 by Brendan Eich, JavaScript was designed to add interactivity and animations to web pages. Over time, JavaScript has undergone significant evolution, with the release of ES14 in June 2023 marking a milestone in its journey. This new version incorporated advanced features and a greater focus on performance optimization and compatibility across various devices, including mobile and IoT.
JavaScript holds a unique place among programming languages. It’s a client-side javascript programming language found in 97.7% of all websites, often used alongside HTML and CSS to produce dynamic and interactive web pages. If you want to learn the javascript programming language, it’s essential to understand the key features that set JavaScript apart, including:
- Its lightweight nature
- Support for dynamic typing
- Object-oriented programming
- Functional style
- Platform independence
It employs an imperative programming paradigm that emphasizes the execution flow of code, in contrast to other programming languages that may utilize different paradigms.
The JavaScript ecosystem is vast and constantly evolving. The language has been instrumental in the development of web applications, from simple websites to complex software. Thanks to its cross-platform capabilities, JavaScript is not confined to the browser. It’s also used server-side through Node.js for developing scalable web servers and back-end infrastructure.
JavaScript’s versatility is further demonstrated by its compatibility with other languages to build high-performance web applications through advancements like WebAssembly. The modern JavaScript ecosystem includes tools like linting, bundling, and transpiling, signalling its critical role in web development.
The Essence of JavaScript
JavaScript is the lifeblood of dynamic web pages. It’s the key to making web pages interactive and engaging, enabling them to interact with visitors and execute complex actions. JavaScript’s core client-side language features allow for storing values in variables, operations on strings, and running code in response to events like clicks. It is used for displaying animations and modifying text visibility. It is also useful for creating dropdown menus.
By validating user input, providing immediate feedback without page reloads, and creating richer interfaces with components like drag-and-drop, JavaScript enhances the user experience in countless ways.
JavaScript's Place in Web Development
JavaScript’s role in web development cannot be overstated. It’s a cross-platform, object-oriented language that adds interactive elements such as dynamically updating content, animations, and clickable buttons to web pages. In addition to its client-side capabilities, JavaScript is also employed server-side through Node.js, enabling the development of scalable web servers and back-end infrastructure.
The .js file extension is the industry standard for JavaScript code, aligning with conventions such as .html for HTML documents, underlining its place in web development.
JavaScript and Web Browsers
Web browsers and JavaScript have a symbiotic relationship. As an interpreted programming language, JavaScript is run directly in text form by web browsers without the need for prior compilation. The sequence of operations in JavaScript is sensitive to the order in which scripts and variables are declared, as web browsers process JavaScript code from top to bottom.
The Document Object Model (DOM) API is integral to JavaScript’s ability to make real-time changes to both the content and style of a web page. This dynamic manipulation of web page content enables JavaScript to provide immediate interaction and feedback within the browser.
In addition to creating dynamic content, controlling multimedia, and animating images, JavaScript offers capabilities beyond the scope of HTML and CSS. Script execution within a web browser can be influenced by attributes like async and defer in the <script> element, which help manage when scripts are run in relation to the page load. Running JavaScript from external files is a common practice that promotes cleaner HTML and code reusability, while also aiding in the organization of the codebase.
Writing Your First JavaScript Code
Now that we’ve set the stage with a solid understanding of what JavaScript is and its role in web development, it’s time to put theory into practice and learn JavaScript. Let’s delve into writing your first JavaScript code.
In this section, we’ll guide you through crafting a basic script, choosing the right text editor, and executing your masterpiece in a browser. We’ll introduce you to the concept of the console.log function, a fundamental tool in the JavaScript developer’s toolkit that is used to print output to the console, serving as a simple way to see your code’s result. We’ll also discuss the importance of comments in JavaScript, which are made with // for a single line or // for multiple lines and are crucial for explaining code logic.
Crafting a Basic Script
Writing a JavaScript program is like writing a story. Each line of code is a sentence, each function is a paragraph, and together, they form a narrative that the computer follows to perform tasks. You start by creating a .js file that can be written in any text editor. The process of writing JavaScript code involves the proper use of semicolons to end lines, enclosing strings with quotation marks, and utilizing comments for developer clarity.
Once you’ve written your script, it’s time to bring it to life. Your script is executed using a web browser or a JavaScript runtime environment like Node.js, allowing you to see the results of your code.
The Text Editor: Your Coding Companion
A text editor is your trusty sidekick on your JavaScript journey. It’s a tool that enhances your coding efficiency through features like:
- Syntax highlighting
- Error-checking
- Automatic indentation
- Auto-completion
These features allow you to easily distinguish different parts of your code, prevent mistakes, and write code faster. Code editors can be expanded with plug-ins for additional functionalities like code snippets, which can help streamline development for specific project needs.
Despite the ability to write JavaScript in basic text editors like Notepad, choosing advanced code editors like Visual Studio Code offers more powerful features for JavaScript developers, including web developers. Mastering a text editor elevates your productivity by providing clarity and consistency in writing code, which aids in learning and applying JavaScript programming concepts.
Running JavaScript in Your Browser
To see the fruits of your labor, you need to run your JavaScript code. You can do this directly in a browser’s console. To open the developer console, right-click on the browser window, select ‘inspect’, and then click on the ‘console’ option. The console operates as a Read Evaluate Print Loop (REPL), where you can write JavaScript code, and execute it, and the browser will display the output.
For executing larger and more complex JavaScript code, it is preferable to write the code in a JavaScript file and link it to an HTML file. The browser can then execute the JavaScript code. By running JavaScript files directly in the browser or linking to an HTML file, you can choose the best method for your coding needs.
Variables and Data Types: Storing Information in JS
As you venture deeper into JavaScript, you’ll encounter two foundational concepts: variables and data types. Understanding JavaScript basics like these will help you progress in your coding journey.
A variable is a name given to a value. It’s like a label on a jar. Just as you might label a jar “sugar” and fill it with sugar, you can create a variable in JavaScript and assign it a value. This allows you to reference and manipulate data in your code.
Variables can have any name that adheres to certain rules:
- They can only include alphabet letters, numbers, underscores
- They cannot begin with a number
- They cannot be a reserved keyword
- They are case-sensitive
Camel case and snake case are two common naming conventions.
Data types in JavaScript are the different kinds of data that variables can hold. These include:
- strings
- numbers
- booleans
- arrays
- objects
- null
- undefined
By understanding variables and data types, you can effectively store, reference, and manipulate data in your code.
Understanding Variables
To declare a variable in JavaScript, you use the keywords ‘var’, ‘let’, or ‘const’, followed by an appropriate name for the variable. Variables act as containers for storing data values and can be assigned values of any data type, allowing for easy data access and manipulation within the code. “Strict mode” in JavaScript, activated by adding ‘use strict’; at the start of a script or function, helps ensure that variables are declared properly, providing cleaner and more secure code.
One of the oddities of JavaScript that often confuses beginners is “hoisting”. Hoisting is a behavior where variable declarations using ‘var’ are lifted to the top of their scope, which is an important behaviour programmer must understand when declaring variables.
Exploring JavaScript Data Types
Now, let’s dive deeper into data types. Think of data types as the various kinds of ingredients you can store in your variable jars. JavaScript supports several primitive data types, including:
- String: used for textual data, and operations on strings create new strings since strings are immutable
- Number: can be integers or floating-point numbers, used for mathematical operations, and are also immutable
- Boolean: represents true or false values and is essential for control flow via conditional statements
- Undefined: signifies an undeclared variable
- Null: represents an intentional absence of value
- Symbol: a unique and immutable data type that is often used as an identifier for object properties
These data types represent single, immutable values.
In addition to these primitive types, JavaScript also has reference data types like:
- Objects
- Arrays
- Functions
- Date
These are collections of data or objects with properties and methods that are mutable. Unlike primitive types, where variables store the actual value, reference type variables hold a reference to the data’s memory location. Hence, when comparing primitive types, their content is checked, but for reference types, the memory address they point to is considered.
Another interesting facet of JavaScript is type coercion, which allows implicit conversion between data types, like treating numbers as strings in certain contexts.
Variable Scope and Use
Scope is another crucial concept in JavaScript. It refers to the area of code where a variable is visible and accessible. A variable declared outside of any function has a global scope and can be accessed anywhere in the program. On the other hand, local scope pertains to variables declared inside a function, meaning they can only be accessed within that function. The keywords ‘let’ and ‘const’ provide block-level scope, reducing the risk of accidental variable leakage outside the intended scope.
The lifespan of a JavaScript variable is determined by its scope. Local variables are deleted after the function they’re declared in has executed, while global variables remain until the program ends or the web browser is closed.
Adding JavaScript to a Web Page
Once you’ve written your JavaScript code, the next step is to add it to a web page. JavaScript can be added to an HTML document either internally within the <script> tag or externally through a separate file. The <script> tag can be placed in either the <head> or the <body> of an HTML document. Its position determines when the JavaScript is executed.
Internal vs. External Scripts
When it comes to adding JavaScript to a web page, you have two main options: internal and external scripts. Internal scripts are written directly within the <script> tag in an HTML document. This method is simple and convenient, but it can make the HTML document cluttered and harder to maintain if the JavaScript code is large or complex.
External scripts, on the other hand, are written in a separate JavaScript file and then linked to the HTML document using the <script src=”filename.js”> tag. This method keeps the JavaScript code organized and allows for its reuse across multiple HTML pages.
Adding JavaScript to a Web Page
Whether you’re using internal or external scripts, the <script> tag plays a crucial role. This tag is used in HTML to embed inline JavaScript code within the <head> or <body> tags or to reference an external JavaScript file using the <script src="filename.js"> element. Using external scripts can lead to better performance for web pages, as browsers can cache the external JavaScript file, resulting in faster page load times during subsequent visits.
The <script> element is commonly used for JavaScript, similar to how the <link> and <style> elements are used for CSS. However, the placement of this tag within the HTML document can affect the loading and execution time of the JavaScript code.
Controlling the Flow: Conditional Statements and Loops
As you delve deeper into JavaScript, you’ll encounter constructs that allow you to control the flow of your code. Conditional statements and loops are powerful tools that enable you to execute selective pieces of code based on specific conditions and automate repetitive tasks.
Conditional statements are like the decision-making bodies of your code. They evaluate a condition and decide which code to execute based on whether the condition is true or false.
Loops, on the other hand, are like the workhorses of your code. They repeat the same task over and over, as long as a certain condition is met.
Making Decisions with If Statements
In JavaScript, the primary tool for making decisions is the if statement. It is written with the if keyword, followed by a condition in parentheses, and a block of code in curly braces that executes if the condition is true. You can also add else if and else blocks to create multiple pathways for code execution, providing alternative code to execute based on varying conditions.
Nested if statements allow for more granular decision-making by evaluating conditions within another if statement’s code block. Logical operators like && (AND), || (OR), and ! (NOT) can be used within if statement conditions to determine the truthiness of combined expressions, enabling complex decision-making.
Automating Tasks with Loops
Loops in JavaScript are all about automation. They repeatedly execute a block of code until a specified condition becomes false. The for loop is a popular type of loop in JavaScript. It’s composed of three main parts: initialization, condition check, and the final expression, which together establish precise control over the number of times code is iterated.
If the number of necessary iterations cannot be determined prior to loop execution, while loops are ideal. They continue as long as a specified condition remains true. A do...while loop guarantees that the loop’s code block is executed at least once by deferring the condition checking until after the first iteration.
Functions: Reusable Blocks of Code
One of the most powerful concepts in JavaScript is the function. A function is a piece of code designed to carry out a specific task. It is a fundamental component of computer programming. Once you’ve defined a function in JavaScript, you can call it multiple times to perform the same task.
Functions can also take parameters, which are inputs that customize the behavior of the function. If a function with parameters is called without passing the arguments, those parameters will take the value of undefined, unless default values are specified.
Creating Your First JavaScript Function
Creating a function in JavaScript is straightforward. You start by using the function keyword followed by a name for the function. Then, you add parentheses that may include parameters, and finally, curly brackets that contain the code to be executed. Once a function is defined, it can be executed by calling its name followed by parentheses, thus running the enclosed block of code.
You can also integrate functions with event handling by triggering called functions upon user interactions like clicks.
Parameters and Return Values
Parameters are what make functions so versatile. They allow the function to accept inputs, which can be used to customize its behavior. Function arguments are the actual values passed to the function when it is called. They act as local variables within the function, without any type of checking performed on them.
If a function is called with missing arguments, the missing values are treated as undefined. The return statement in a JavaScript function is used to pass a value back to the function’s caller, and a function may have multiple return points.
Arrow Functions: A Modern Twist
Arrow functions offer a more concise syntax for writing functions. They’re defined using the => arrow notation rather than the traditional function keyword. Arrow functions are advantageous for their brevity and the fact that this keyword inside an arrow function refers to the context in which the function was defined, which can differ significantly in behavior from traditional function expressions.
Interacting with Web Pages: The Document Object Model (DOM)
The DOM is a programming interface for web documents that represents the page so programs can change the document structure, style, and content. JavaScript allows for dynamic modification of HTML and CSS through the DOM to update the user interface.
What is the DOM?
The DOM models a document as a hierarchy of nodes and objects, which allows languages like JavaScript to interact and manipulate the page dynamically. JavaScript is most commonly used with the DOM in web development to create interactive web pages by manipulating the content, structure, and style.
Selecting and Modifying HTML Elements
With JavaScript and the DOM, you can take full control of the elements on a web page. You can select HTML elements using methods.
Once selected, you can manipulate these elements using properties and functions like Element.innerHTML for content editing, Element.appendChild to add new child elements, and Node.removeChild to remove existing child nodes, along with functions for setting and getting attributes.
Event Handling: Responding to User Actions
Event listeners can be added to HTML elements using JavaScript to make web pages responsive to user interactions. These event listeners are basically like attentive butlers, waiting for a specific signal (or "event") to spring into action and execute a block of code.
For example, when a user clicks a button, hovers over a link, types into a form field, or presses a key, these interactions can trigger events. JavaScript provides a variety of event types such as 'click', 'mouseover', 'input', and 'keydown', allowing developers to tailor responses to a wide range of user behaviors.
Adding an event listener is quite simple. You select an element, call the addEventListener method on it, and pass two arguments: the name of the event and the function to call when the event occurs. Here's a basic syntax example:
javascript element.addEventListener('click', functionToCall);
The functionToCall can either be a named function or an anonymous function defined right in the call. This flexibility allows for both reusable and one-off event response strategies.
Moreover, event listeners can be removed using the removeEventListener method, providing control over when your web page should stop listening for specific events. This is particularly useful for optimizing performance and managing user experience.
In essence, event handling in JavaScript is a powerful mechanism that adds a layer of interactivity to web pages, making them not just static displays of content but dynamic interfaces that can engage and respond to users in real time.
Embracing JavaScript for Interactive Web Experiences
JavaScript stands as a cornerstone of web development, integral to creating interactive and dynamic user experiences. With its versatile capabilities ranging from simple page enhancements to complex web applications, JavaScript empowers developers to build rich internet applications that are both engaging and accessible.
As we have explored the various facets of JavaScript, from its syntax and functions to its interaction with the DOM and event handling, it is evident that mastering JavaScript is key to modern web development. Whether you are just starting out or looking to deepen your understanding, the journey of learning JavaScript is one of continuous discovery and innovation.
For those who are eager to accelerate their learning curve, Nexacu's courses offer a structured path to mastering JavaScript. Nexacu, a leader in tech training, provides comprehensive courses that cover everything from the basics to advanced topics. Our expert instructors guide learners through hands-on exercises and real-world projects, ensuring that students not only understand the concepts but can also apply them effectively.
By enrolling in Nexacu's JavaScript courses, developers can enhance their skills in a collaborative learning environment. These courses are designed to help you write code confidently, experiment with new features, and stay updated with the latest developments in the JavaScript ecosystem. As a result, you will grow as a developer and harness the full potential of JavaScript to create captivating web experiences.
Frequently Asked Questions (FAQs)
What is JavaScript and why is it important?
JavaScript is a high-level, interpreted programming language that is primarily used for creating dynamic and interactive web pages. It is an essential component of web development, alongside HTML and CSS, and allows developers to add behavior to web pages, including handling events, creating animations, and managing user input. JavaScript's importance lies in its ubiquity across the web and its role in enhancing user experience.
Can JavaScript be used for server-side programming?
Yes, JavaScript can be used for server-side programming, thanks to platforms like Node.js. Node.js is a JavaScript runtime that enables developers to write server-side scripts and create back-end infrastructure using JavaScript. This has expanded the capabilities of JavaScript beyond the browser, allowing for the development of full-stack applications with a single programming language.
How do I add JavaScript to my web page?
JavaScript can be added to a web page either inline, by writing code directly within <script> tags in an HTML document, or externally, by linking to a separate .js file. External JavaScript files are linked using the <script src="filename.js"> tag. The placement of the <script> tag within the HTML document can affect when the JavaScript code is executed.
What are some common tools and frameworks used with JavaScript?
The JavaScript ecosystem includes a variety of tools and frameworks designed to assist in development and improve workflow. Some common tools include code linters, bundlers, and transpilers, while popular frameworks and libraries include React, Angular, Vue.js, and jQuery. These tools and frameworks provide pre-written code and functionalities that simplify the process of building complex applications.
Is JavaScript the same as Java?
No, JavaScript and Java are two distinct programming languages with different syntax, concepts, and uses. JavaScript is primarily used for web development to create interactive web pages, whereas Java is a general-purpose programming language that can be used for a wide