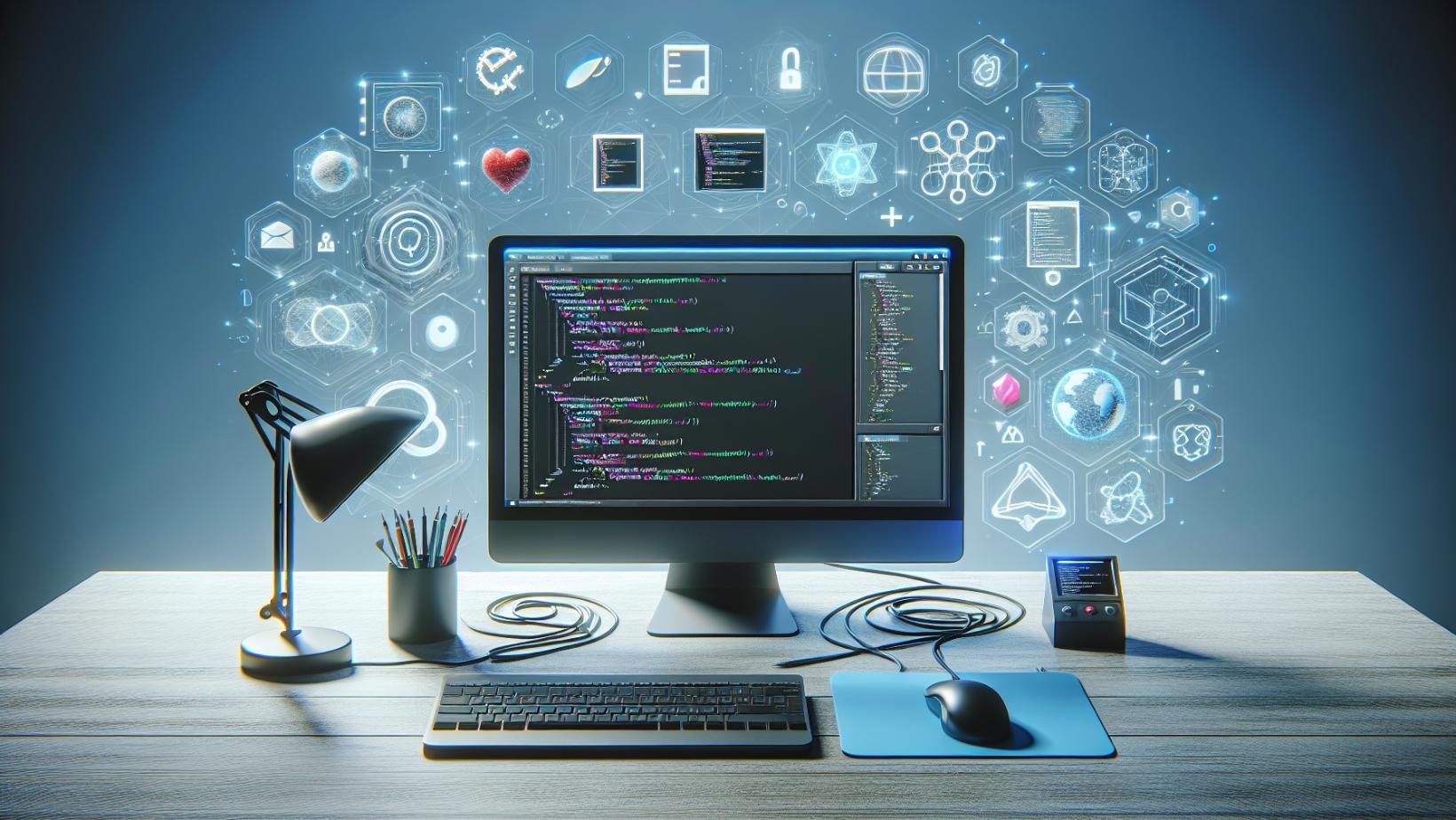
JavaScript is the language that powers the web. Whether you’re just starting out or looking to deepen your expertise, understanding JavaScript is key to building dynamic, interactive websites. This guide will take you through the core concepts, from basic syntax to advanced functions, and show you how JavaScript interacts with HTML, CSS, and APIs to create seamless user experiences. Ready to dive into the world of JavaScript? Let’s get started on the path to becoming a proficient JavaScript developer in modern web development.
Key Takeaways
-
JavaScript is a versatile and essential programming language for interactive web development, influencing many aspects of modern internet technology, including dynamic behavior, web scripting, and its evolution from a simple scripting language to a robust tool for building complex applications.
-
A strong understanding of JavaScript basics, including writing scripts, variable manipulation, and control flows, is necessary for developing responsive user interfaces, and mastering more advanced concepts like asynchronous operations and server-side development with Node.js allows for the creation of more sophisticated applications.
-
JavaScript’s integration with HTML, CSS, APIs, and emerging technologies like WebAssembly enables developers to build seamless and high-performance web applications, while staying current with the latest trends and tools ensures developers can continue to deliver innovative web solutions.
Exploring the JavaScript Language
At the heart of modern web development lies JavaScript, an essential programming language that breathes life into static web pages. Imagine a world where web pages react to every click, swipe, and keystroke – that’s the power of JavaScript at work. As a high-level, multi-paradigm language, JavaScript’s syntax is influenced by C and Java, yet it maintains its unique flexibility. It features a rich set of types, operators, and control structures, enabling dynamic behavior and interactivity on web pages.
For those beginning a JavaScript course or seeking to refine their javascript skills, mastering the fundamental aspects of the language proves invaluable for programming sophisticated, responsive user interfaces.
In this course, you will:
-
Uncover the structure of JavaScript code
-
Delve into its web scripting capabilities
-
Observe its transformative journey from an interpreted language to one that significantly influences the modern internet.
The Anatomy of JavaScript Code
Embarking on a journey to learn JavaScript, one must first comprehend the structure of its code. The anatomy of JavaScript code, including the use of code block, is the blueprint for all the magic that happens within the browser window. As a case-sensitive language, JavaScript requires meticulous attention to detail in variable naming and function calls. The syntax is the skeleton upon which logic is built, mandating semicolons to terminate statements and quotation marks to encase strings.
Variables, the vessels that hold data, are declared with precision using keywords like ‘let’ and manipulated with assignment operators. For aspiring developers in the world of JavaScript, it’s essential to comprehend the subtleties of code blocks, ranging from string concatenation to appreciating the importance of types and values.
Scripting for the Web
JavaScript is the puppet master of web pages, pulling the strings of the Document Object Model (DOM) to orchestrate a symphony of interactive elements. Scripting for the web is about embedding JavaScript source code into HTML, strategically placing scripts to ensure seamless execution. Through methods like querySelector(), JavaScript can manipulate HTML elements, while event handlers respond to user actions with precision. Careful management of user inputs is vital. JavaScript vigilantly maintains the web page’s functionality, even when a user cancels an input prompt.
It’s these meticulous scripts that enable dynamic displays, turning a static page into a thriving hub of activity.
Evolution of JavaScript
The tale of JavaScript’s evolution is one of innovation and adaptation. From its inception as Mocha to its present-day stature, JavaScript has undergone a metamorphosis, aligning with the ever-changing landscape of web technologies. The original JavaScript, crafted to complement the robustness of Java, quickly became the linchpin for interactive web development. The language has braved the tides of time, from the browser wars that led to the creation of JScript to the standardization efforts that culminated in ECMAScript.
Today’s JavaScript is a testament to its resilience and capability, continually embracing new features and fostering a prolific ecosystem of libraries and frameworks that propel it forward.
Getting Started with JavaScript Basics
Moving from the wider aspects of the language to the intricate details, the journey to master JavaScript basics is akin to traversing a thousand lines of code. It starts with writing your very first script and evolves into a deep understanding of variables, data types, and the logic that directs the flow of execution. The beauty of JavaScript lies in its ability to execute across all major web browsers, making it a universal tool for budding developers.
Commencing your JavaScript course, bear in mind that understanding the basics is the cornerstone to erecting a sturdy foundation for more intricate structures.
Writing Your First JavaScript Script
Visualize crafting your inaugural JavaScript script - a significant landmark in every developer’s journey. By embedding JavaScript code within an HTML file using the <script> tag, you open a door to endless possibilities. A simple script can alter the web page, whether it’s by outputting text to the console or changing the content viewers interact with.
The moment you open that HTML file in a browser, your code comes to life, ready to be tested and tweaked. This is where the adventure begins, where the first line of JavaScript you write paves the way for countless more.
Understanding Variables and Data Types
In the world of JavaScript, variables are the chameleons of code, changing types with a programmer’s whim. The dynamic typing of JavaScript allows for a flexible approach to programming, but with great power comes great responsibility. Developers must navigate the nuances of type conversions to prevent unintended errors.
Be it manipulating strings, processing numbers, or managing booleans, grasping the nature of JavaScript data types is crucial. Using the typeof function, one can become a type detective, ensuring that variables are exactly what they need to be at any given moment in execution.
Control Flow Mastery with If Statements
Control is the name of the game when it comes to programming, and JavaScript’s if statements are the central players. These conditional constructs serve as the decision-makers, guiding the flow of execution based on specified criteria. By mastering the use of if, else, and logical operators, developers can create complex, logic-driven scripts that respond intelligently to different scenarios.
The correct syntax and structure are the keys to harnessing the full potential of if statements, turning the unpredictable nature of user input into a seamless experience that feels both responsive and intuitive.
Diving Into Functions and Scopes
Venturing further into JavaScript’s domain, functions and scopes become crucial, presenting a modular method for code organization and execution. Functions are the building blocks of JavaScript applications, empowering developers to encapsulate code for reuse, while scopes determine the accessibility of variables. It’s in understanding these concepts that one can craft sophisticated applications capable of maintaining state across multiple calls and embracing the power of closures.
As we wade through JavaScript basics, it becomes clear that functions and their associated scopes are pivotal in crafting clean, efficient, and maintainable code.
Crafting a Simple Function
Crafting a simple function in JavaScript is akin to writing a new chapter in your application’s story. It begins with the ‘function’ keyword, a name, and then a set of parentheses that may enclose parameters – an open invitation for data to come and play. Within the curly braces lies the function’s soul, a block of code ready to execute upon invocation.
Imagine a function that adds two numbers; it’s a simple task, but it encapsulates the essence of JavaScript’s power to perform operations and return results. Invoking a function is the final step in its journey, a call to action that breathes life into the logic within.
Arrow Function and Anonymous Function Use Cases
Arrow functions and anonymous functions find their use cases in the elegant tapestry of JavaScript code, each offering distinctive benefits. Arrow functions, with their concise syntax, are particularly well-suited for situations where the ‘this’ context of the surrounding code is critical, such as in callbacks and promises.
Anonymous functions, on the other hand, are the stealth operatives of JavaScript, often utilized in scenarios that demand temporary functionality without the need for a name. Understanding when to employ these functions is a mark of a skilled JavaScript developer, one who recognizes the right tool for the task at hand.
Variable Scope and Hoisting
The concepts of variable scope and hoisting in JavaScript are akin to understanding the laws of a secret society. Variables declared with ‘var’ live within the function or out in the open as globals, while ‘let’ and ‘const’ prefer the seclusion of blocks. The curious behavior of hoisting, where declarations are lifted to the top of their scope before execution, can lead to unexpected results if not properly understood.
This nuanced dance between scope and hoisting can either be a developer’s ally or foe, and mastering it is essential for creating predictable and bug-free code.
Advanced JavaScript Concepts
As one’s JavaScript journey progresses, the path leads to more advanced territories. Here, concepts like asynchronous operations, functional programming, and the realm of server-side JavaScript await exploration. The language’s ability to adapt is showcased through features like async/await, which simplify complex operations into readable and manageable code.
The advent of functional programming patterns in JavaScript has given rise to pure functions and higher-order functions, transforming the way developers think about and handle data. Advanced JavaScript concepts are not just for the seasoned coder; they’re stepping stones for any aspiring developer, opening doors to more efficient, scalable, and sophisticated web applications.
Asynchronous JavaScript: Callbacks to Promises
The evolution of asynchronous JavaScript is a story of overcoming complexity with elegance. In the early days, callbacks were the primary means of handling tasks like I/O operations or API calls, but they often led to a tangled mess colloquially known as “callback hell.” Promises emerged as the saviors, encapsulating the eventual success or failure of an asynchronous operation in an object, ushering in a new era of chained methods that improved both readability and error handling.
The async/await syntax further refined the landscape, allowing developers to write asynchronous code that feels synchronous, simplifying the flow and making the codebase more intuitive.
Working with Objects and Prototypal Inheritance
In the heart of JavaScript lies its object-oriented nature, where objects and prototypal inheritance form the core of the language’s structure. Objects in JavaScript are dynamic collections of properties, with constructor functions laying the groundwork for defining and initializing these properties. Prototypal inheritance allows objects to inherit features from other objects, enabling developers to create a network of objects that share methods, thereby conserving memory and maintaining a clean codebase.
Understanding the intricacies of object creation patterns and prototypal inheritance opens up a world of possibilities for creating complex data structures and efficient applications.
Server-Side JavaScript with Node.js
Node.js has revolutionized the way developers think about JavaScript, taking it from the confines of the browser and into the vast expanse of server-side development. With Node.js, JavaScript can now build scalable and high-performance applications that run on servers, interact with databases, and manage server tasks directly. It’s an environment that thrives on non-blocking I/O and an event-driven model, making it ideal for real-time applications and APIs.
Node.js not only expands JavaScript’s reach beyond the browser but also unifies development paradigms, allowing developers to use a single language across the entire web development stack.
Essential JavaScript Tools for Developers
Arming oneself with the appropriate tools is vital for any craft, with JavaScript development being no different. From powerful Integrated Development Environments (IDEs) that offer advanced code completion and integrated version control, to simple code editors that boast speed and extensibility, the tooling ecosystem around JavaScript is vast and varied. These tools not only enhance productivity but also provide a nurturing environment where code can be written, tested, and debugged efficiently.
Irrespective of whether you’re commencing your initial JavaScript course or you’re an experienced developer aiming to optimize your workflow, comprehending and leveraging these crucial tools is vital to becoming a skilled JavaScript coder.
Integrated Development Environments (IDEs) and Code Editors
The choice of an Integrated Development Environment (IDE) or code editor can significantly impact a developer’s efficiency and comfort while coding in JavaScript. These environments range from lightweight text editors like Atom and Sublime Text, which offer quick editing capabilities and rich plugin ecosystems, to full-fledged IDEs like Visual Studio Code and WebStorm that provide comprehensive features such as debugging tools and intelligent code assistance.
The decision often boils down to personal preference and project requirements, but one thing remains constant: a good coding environment can make JavaScript development a more pleasurable and productive experience.
Debugging JavaScript
The art of debugging is as crucial as the act of writing JavaScript code itself. With browser developer tools, developers can step into the world of their code, examining it under a microscope to identify and rectify issues. Tools like Chrome’s DevTools or Firefox’s Developer Edition provide a console for logging and sophisticated features to monitor performance, all designed to make debugging less of a chore.
The ‘console.log()’ function has become a developer’s best friend, offering a simple way to track the application’s state, while breakpoints allow for a deeper dive into the code’s execution. Debugging becomes less daunting with these powerful tools, ensuring that JavaScript applications run smoothly and efficiently.
Version Control for Your JavaScript Projects
Version control systems like Git are the backbone of modern software development, including JavaScript projects. They allow developers to track changes, collaborate efficiently, and deploy code with confidence. Platforms such as GitHub offer a place to store code remotely, while the Gitflow workflow provides a robust framework for managing features, releases, and hotfixes.
Best practices such as continuous integration, code reviews, and rigorous testing are enforced through these systems, ensuring that JavaScript projects maintain high code quality. With the right version control strategies in place, developers can focus on innovation rather than worrying about codebase integrity.
Enhancing Web Pages with JavaScript Interactivity
Highly engaging web pages are characterized by their elegant and agile response to user interaction, an arena where JavaScript excels. By enhancing web pages with interactive forms, dynamic content, and animations, JavaScript opens up a myriad of possibilities for web developers to captivate their audience.
Whether it’s a simple form validation or an elaborate game, JavaScript provides the tools to create a rich user experience. Understanding how to wield these tools effectively is essential for any developer looking to create web pages that are not just informative but truly interactive.
Manipulating the Document Object Model (DOM)
The Document Object Model (DOM) is the stage upon which JavaScript performs its interactive masterpieces. By manipulating the DOM, developers can:
-
Dynamically update content
-
Change styles
-
Respond to user events
-
All in real-time
JavaScript’s ability to select and modify elements allows for a high degree of control over the user interface, making web pages more responsive and engaging.
Through events like clicks and keystrokes, JavaScript listens and reacts, providing users with an experience that feels both fluid and intuitive. This dynamic manipulation is at the core of modern web development, transforming static HTML into a living, breathing ecosystem.
Creating Dynamic Forms and User Input Validation
Interactive forms are the cornerstone of user engagement on the web, and JavaScript is the artisan crafting their functionality. With JavaScript, forms become dynamic, capable of adding or removing fields in response to user actions and providing real-time feedback through input validation. This not only enhances the user experience but also ensures that the data collected is accurate and useful.
JavaScript’s ability to handle user input with finesse, from simple text fields to complex validation rules, is what makes it an indispensable tool for web developers.
Animations and Transitions
Animations and transitions are the visual spices that give web pages their flavor, and JavaScript is the chef in charge. By leveraging JavaScript, developers can create animations that enhance storytelling, direct user attention, and make interfaces more intuitive. The requestAnimationFrame method allows for smooth animations that synchronize with the browser’s refresh rate, providing an optimal user experience.
JavaScript’s control over the timing and sequencing of animations means that developers can create complex, choreographed experiences that dazzle and delight. It’s this dynamic behavior that can turn a good web page into a great one.
Bridging JavaScript with Other Web Technologies
JavaScript isn’t an isolated entity; it’s a component of a larger web technologies ecosystem that collaborates to generate the enriched web experiences we’ve become accustomed to. By bridging JavaScript with:
-
HTML
-
CSS
-
APIs
-
WebAssembly
Developers can create applications that are not only powerful but also seamless across different platforms and devices. This synergy between JavaScript and other web technologies is what allows for the development of complex web applications, ranging from single-page applications to full-fledged web services.
As developers look to the future, understanding how JavaScript integrates with other technologies will be key to pushing the boundaries of what’s possible on the web.
Integrating JavaScript with HTML and CSS
The trinity of web development – HTML, CSS, and JavaScript – work together to create web pages that are more than just a collection of text and images. JavaScript serves as the connecting thread, bringing interactivity to the structural HTML and stylized CSS. From manipulating the DOM to dynamically adjusting styles, JavaScript enables developers to create interfaces that are both dynamic and responsive to user actions.
This integration is fundamental to modern web development, allowing developers to build web pages that are not only visually appealing but also functionally robust.
Communicating with APIs and Web Services
In today’s interconnected digital landscape, JavaScript’s ability to communicate with APIs and web services is indispensable. Whether it’s fetching data from a server or sending user input to a backend service, JavaScript acts as the intermediary, translating and shuttling data between the client and the server. This communication enables web applications to integrate with external services, enriching the user experience with features like social media sharing, maps, and payment systems.
By mastering API communication with JavaScript, developers unlock a world of possibilities, expanding the functionality of their web applications beyond the confines of a single page.
JavaScript and WebAssembly
WebAssembly is the new kid on the block, and it’s quickly becoming JavaScript’s best ally in pushing the web’s performance boundaries. This low-level binary format allows code written in languages like C, C++, and Rust to run on the web at near-native speeds, providing a performance boost for computationally intensive tasks.
JavaScript and WebAssembly can work in tandem, with JavaScript handling the DOM and WebAssembly crunching the numbers. The result is a powerful combination that enables high-performance applications to run in the browser, further solidifying JavaScript’s role as the lingua franca of the web.
The Future of JavaScript: Trends and Predictions
The future of JavaScript holds as much excitement as its history. Bolstered by an active community of developers and a consistent influx of fresh frameworks and tools, JavaScript persistently remains at the vanguard of web development innovation. The language is evolving to meet the challenges of modern web applications, with trends like server-side rendering, static site generation, and the integration of AI and machine learning.
As new paradigms like the Metaverse and Web3 take shape, JavaScript will undoubtedly play a pivotal role in shaping these digital experiences. By staying abreast of these trends and predictions, developers can future-proof their skills and continue to create cutting-edge web applications.
Summary
From its humble beginnings to its current status as the backbone of the web, JavaScript has come a long way. Throughout this guide, we’ve explored the language’s syntax, delved into its core concepts, and examined its role in both client-side and server-side development. We’ve also highlighted the essential tools that empower developers to write, debug, and manage JavaScript code effectively. As we conclude, it’s clear that JavaScript’s versatility and adaptability will continue to make it a critical skill for developers. By mastering JavaScript, you equip yourself with the tools needed for modern web development, ready to tackle the challenges and opportunities that the future holds.
Frequently Asked Questions
How to start JavaScript for beginners?
To start learning JavaScript as a beginner, consider taking a course, learning from books, attending coding bootcamps, building projects, participating in meetups, and exploring open source projects. These are effective ways to gain a strong foundation in JavaScript.
How do I install JavaScript?
To install JavaScript, you need to activate it in your browser settings by following these steps in Google Chrome: Click on the three-dot menu, go to Settings, then Privacy and Security, click on Site settings, and finally select "Sites can use JavaScript".
Can I download JavaScript for free?
Yes, you can download JavaScript for free from various sources such as complete scripts and libraries that are available for free use.
What is JavaScript are used for?
JavaScript is a programming language used to create dynamic content for websites, allowing for interactive and user-friendly features such as animations, pop-up menus, and multimedia control. It is a versatile tool for enhancing web development skills.
What is JavaScript and why is it important for web development?
JavaScript is essential for web development because it enables interactive web pages and applications, allowing developers to create dynamic user interfaces and improve user experience.